Flow Nodes Scripts
Scripts a written in JavaScript.
A script can wire connections and return values.
There are two ways to write scripts.
There is the script node and then there are variable property scripts.
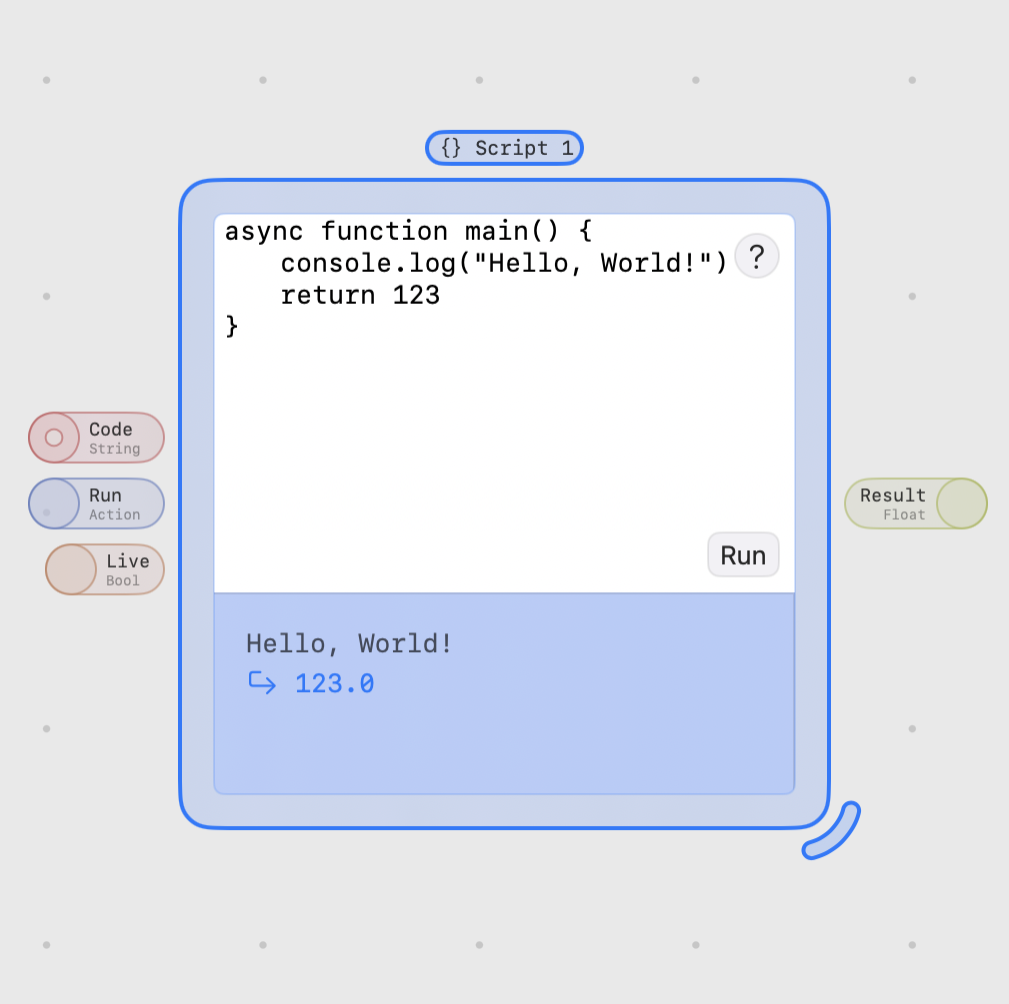
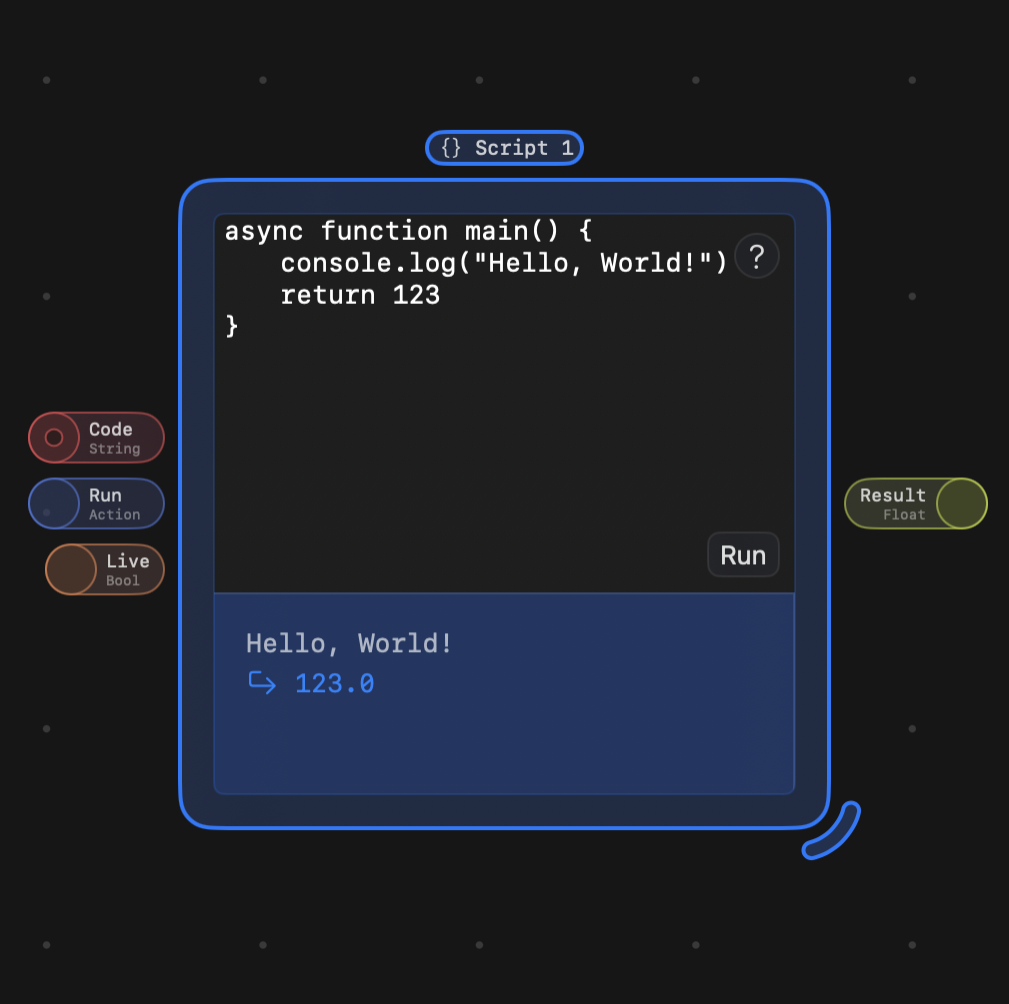
run
manually or set to live
mode, where it will run as soon as one of it's connected wires updates.
Main Script
async function main() { console.log("Hello, World!") return 123 }
This script is composed of a async main function. The script first prints a "Hello, World!"
then returns a number represented as a Float
.
Variable Property Scripts
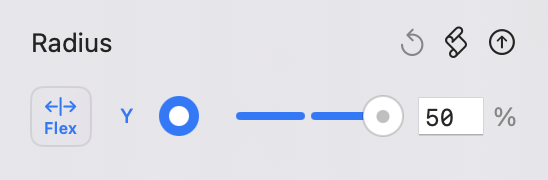
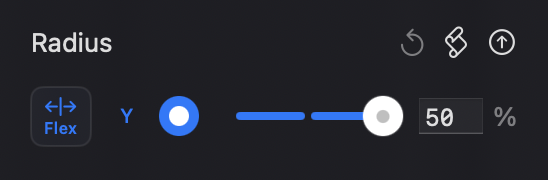
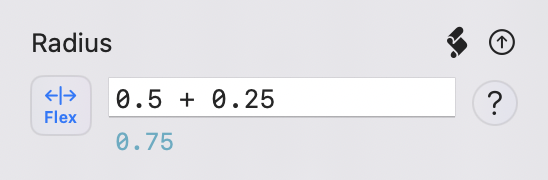
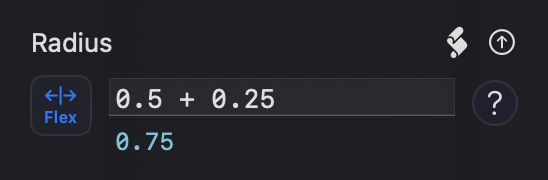
There is a script button on all properties that are "variables", meaning the have a property and an inlet. When in script mode, code can be written to calculate values.
Auto Wire Connections
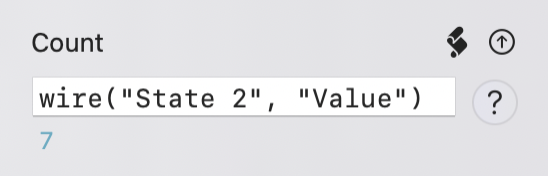
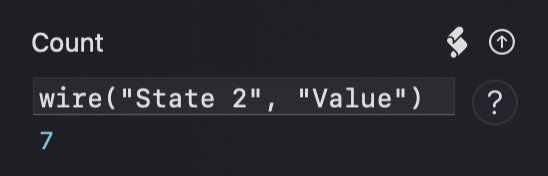
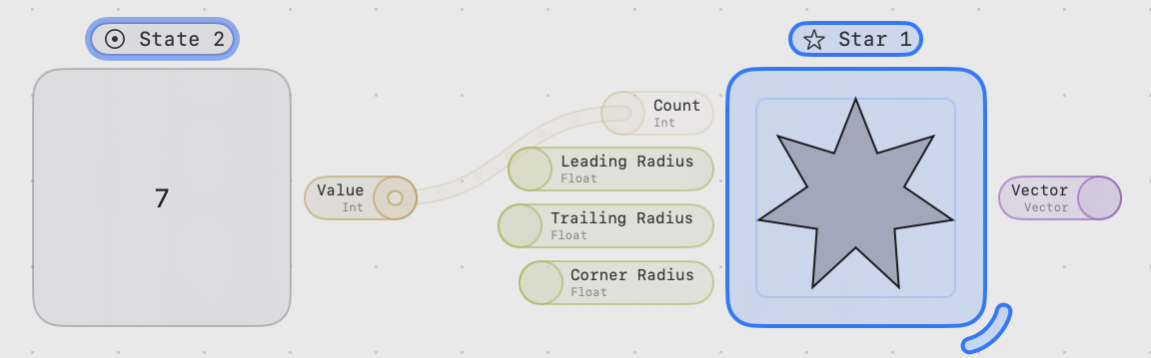
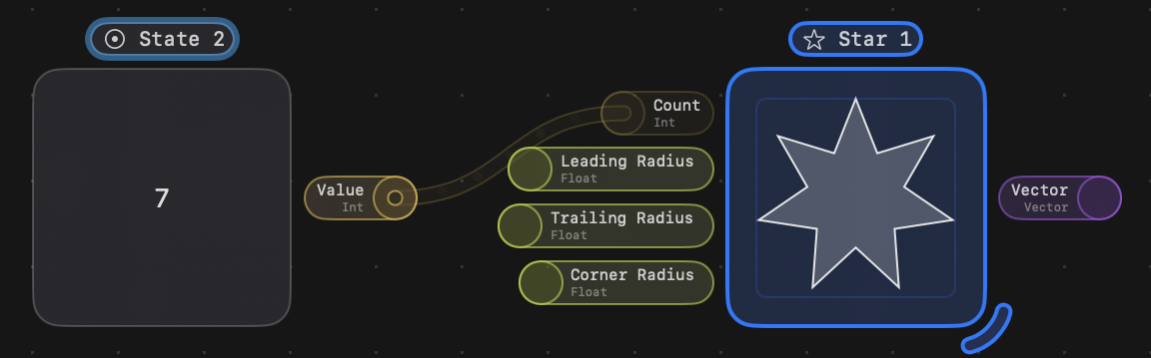
With the use of the wire
function, connections are automatically made. The first argument is the node name and the second argument is the outlet name.
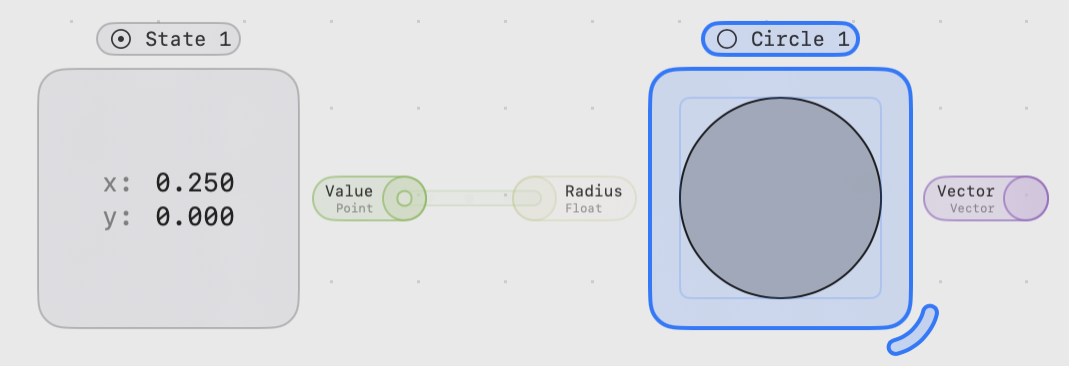
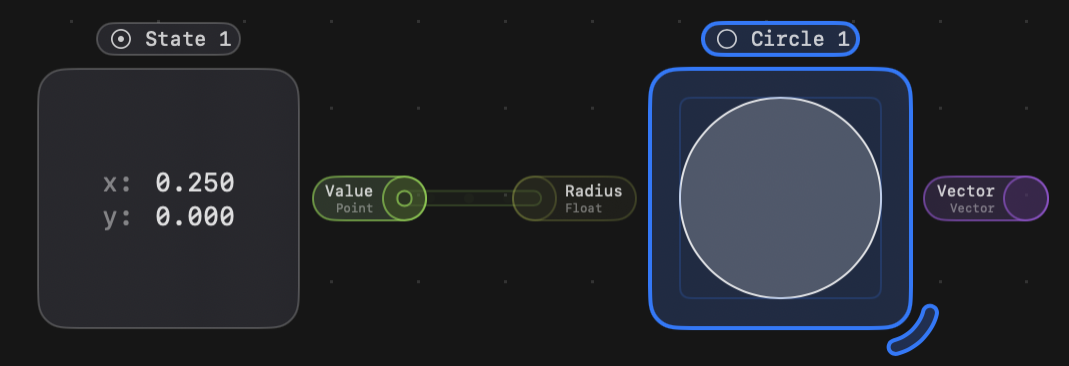
(await wire("State 1", "Value")).x * 2.0
To get sub values, like x or y from a point. You first need to await the wire value.
Script Node
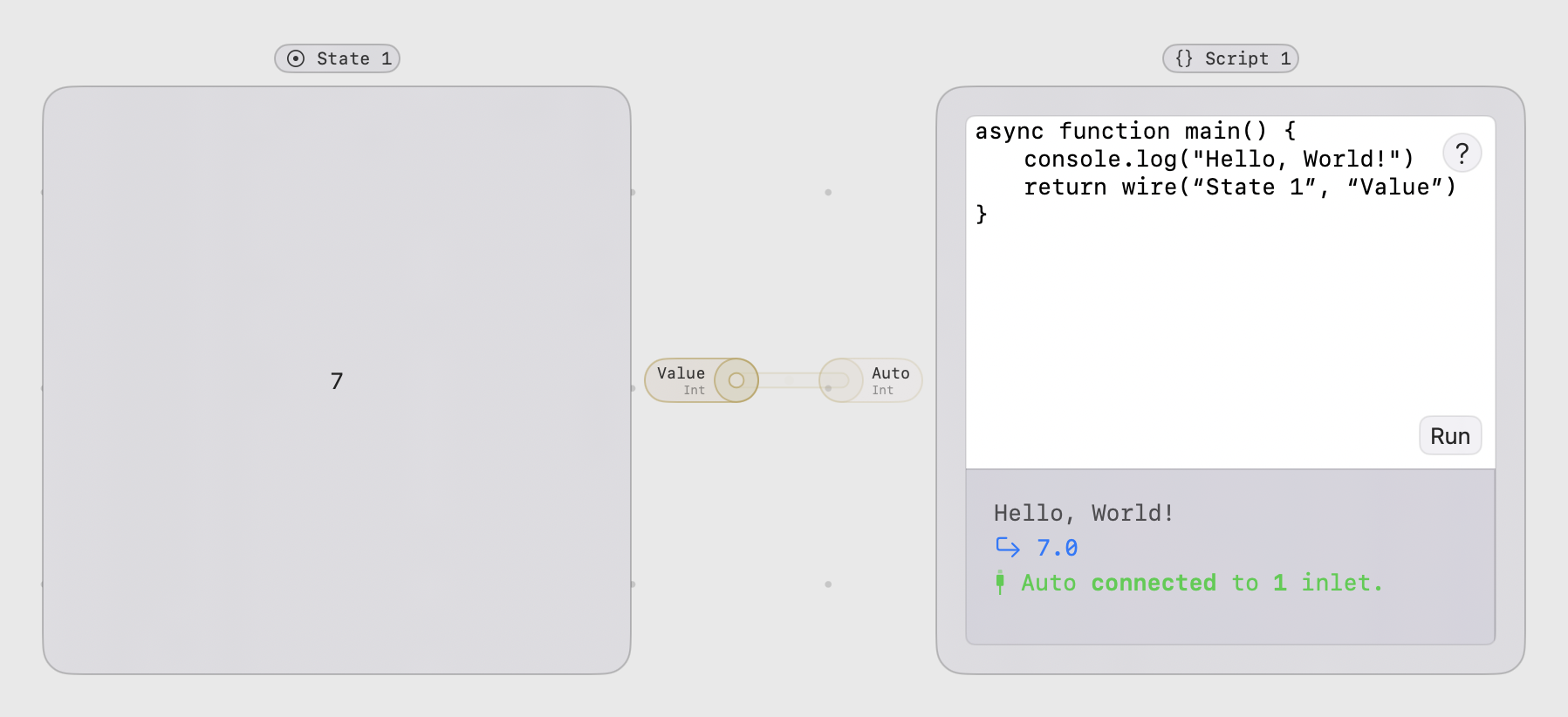
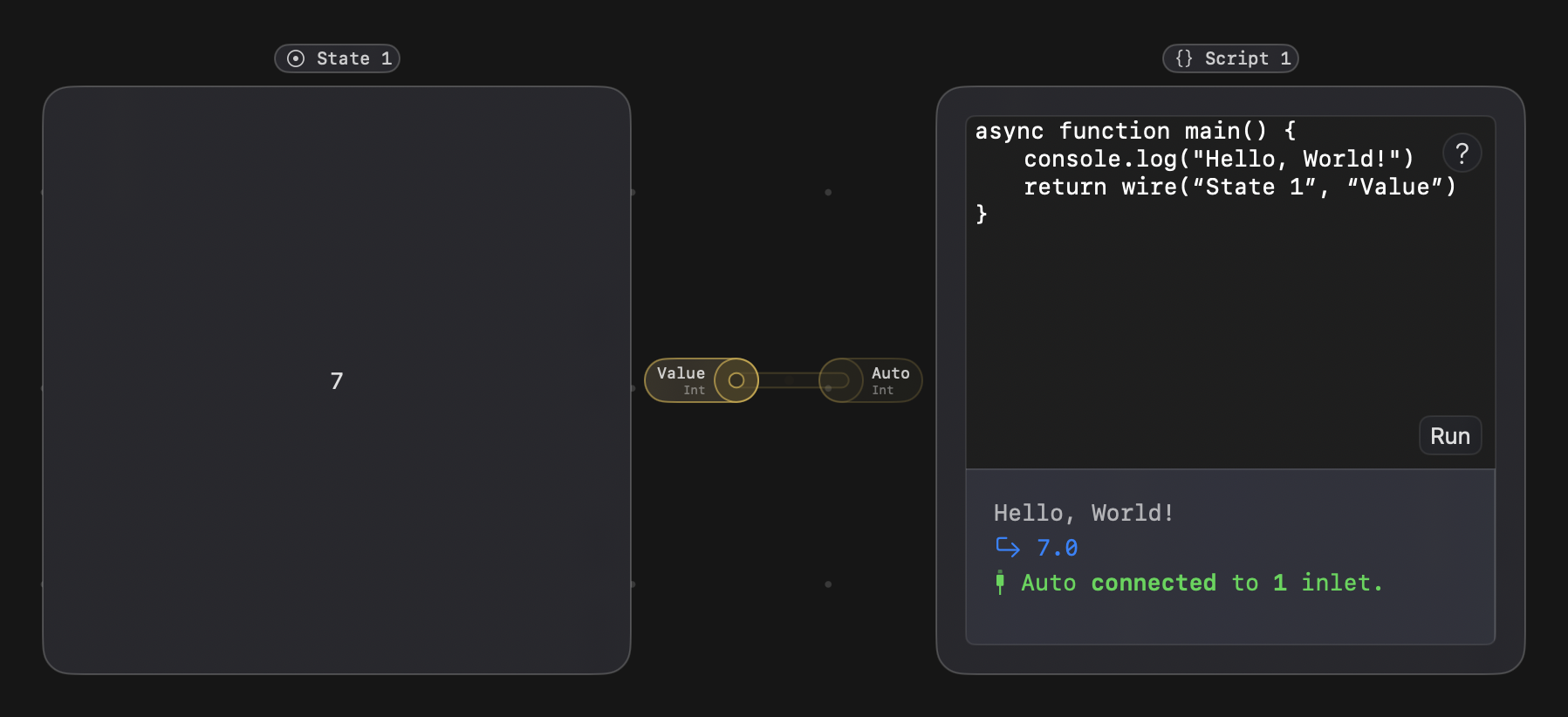
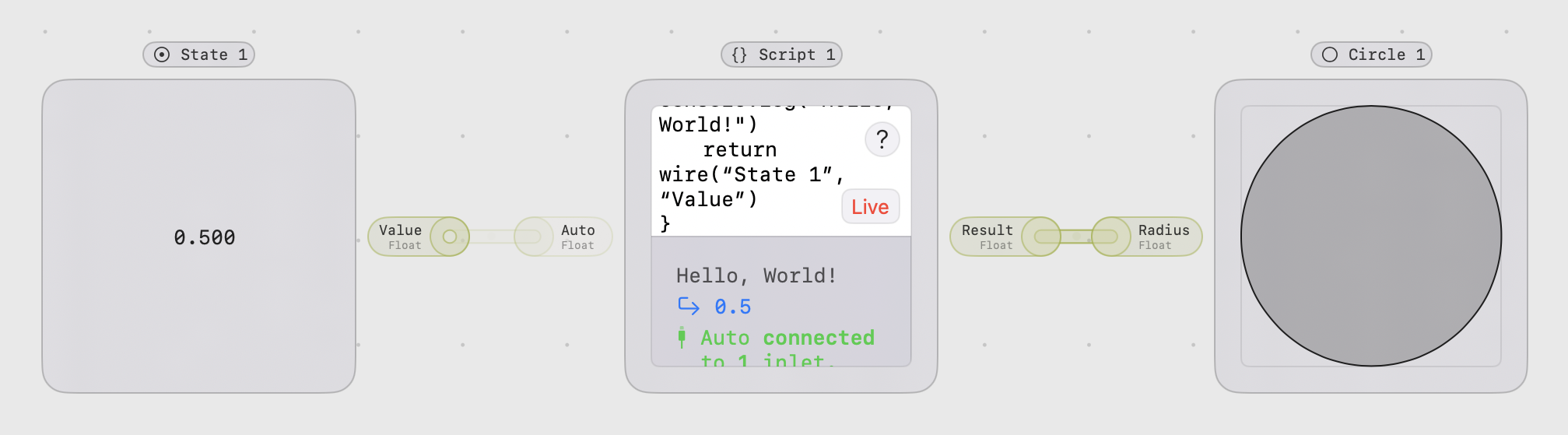
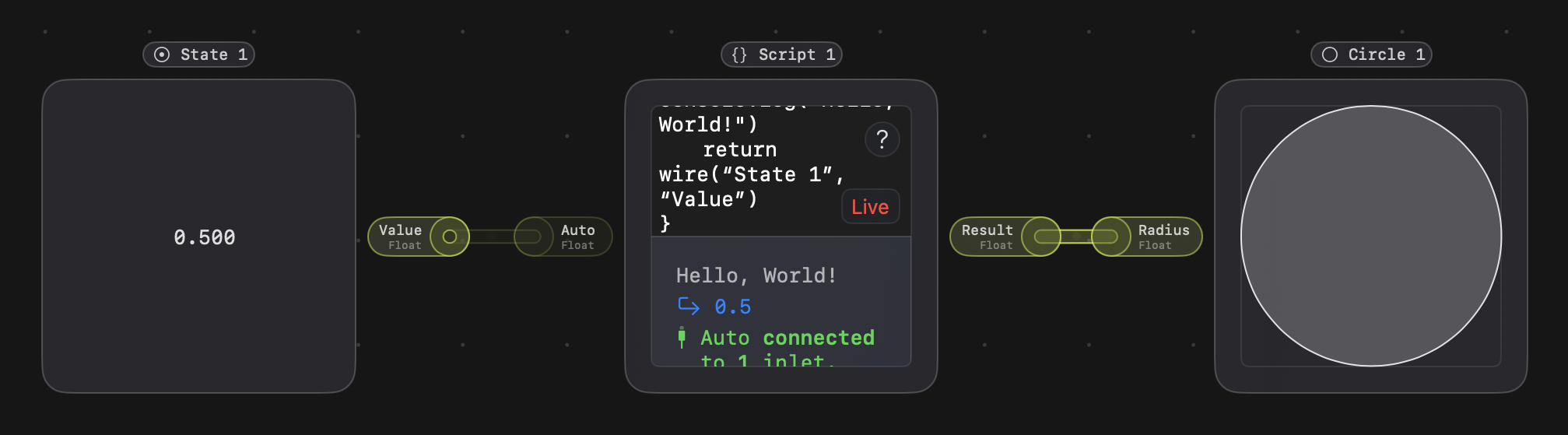
async function main() { console.log("Hello, World!") return wire(“State 1”, “Value”) }
Wires will be auto connected with the wire function.
Values
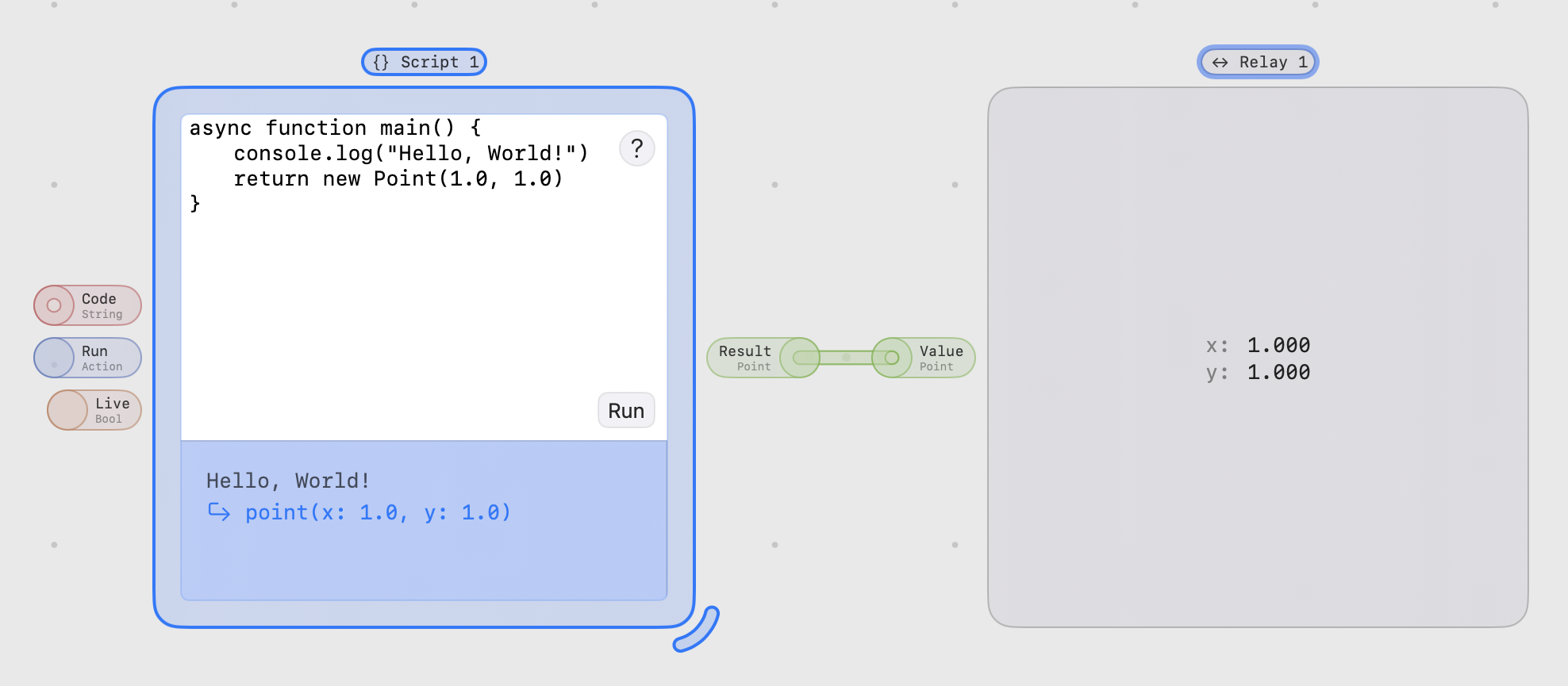
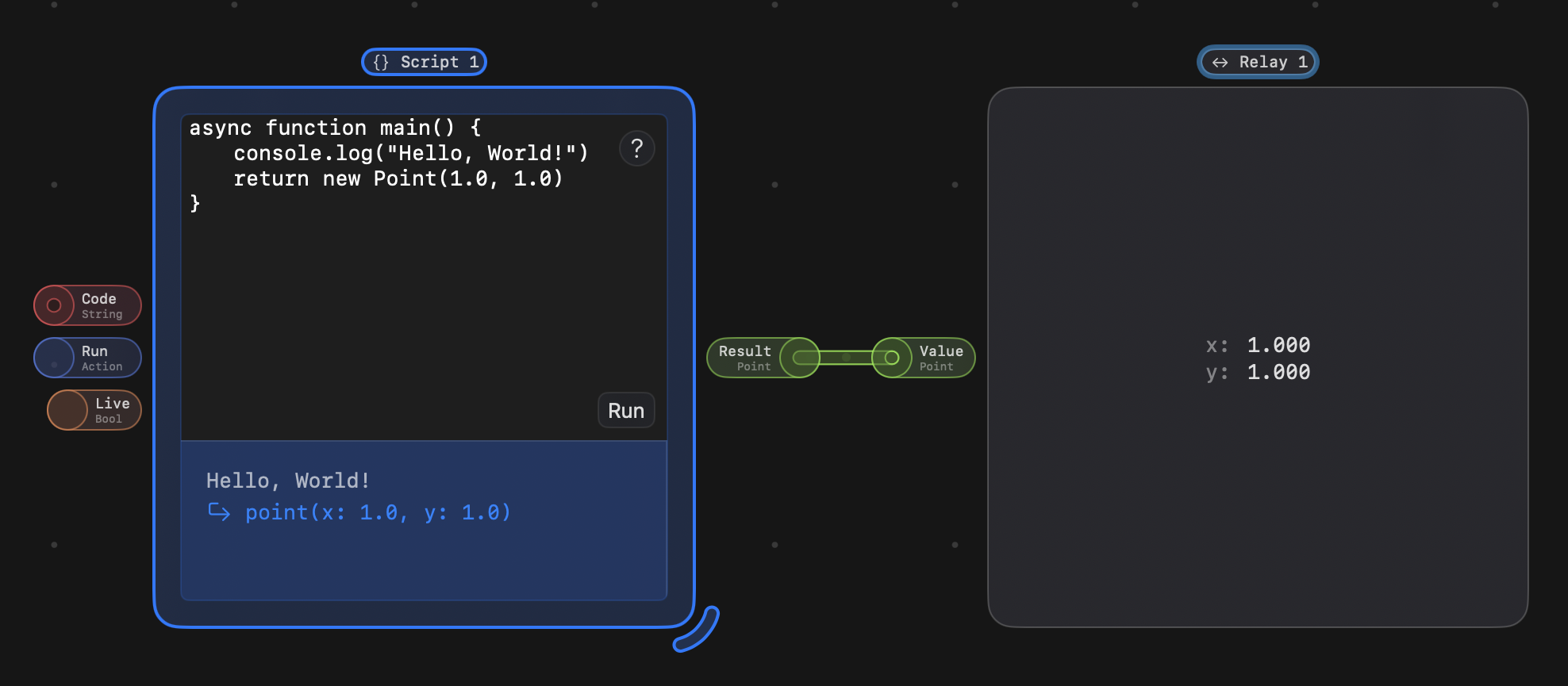
async function main() { console.log("Hello, World!") return new Point(1.0, 1.0) }
Points and other types can be created and returned.
Loops
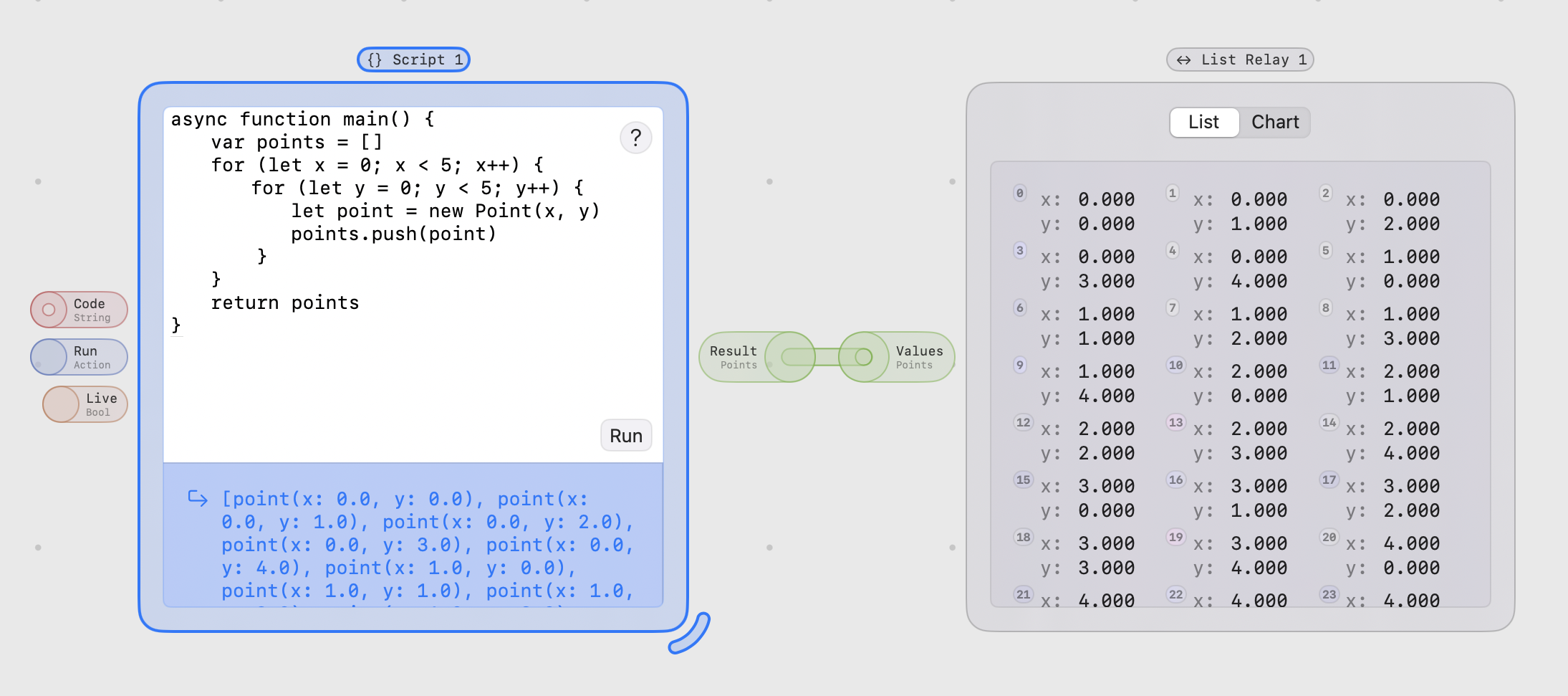
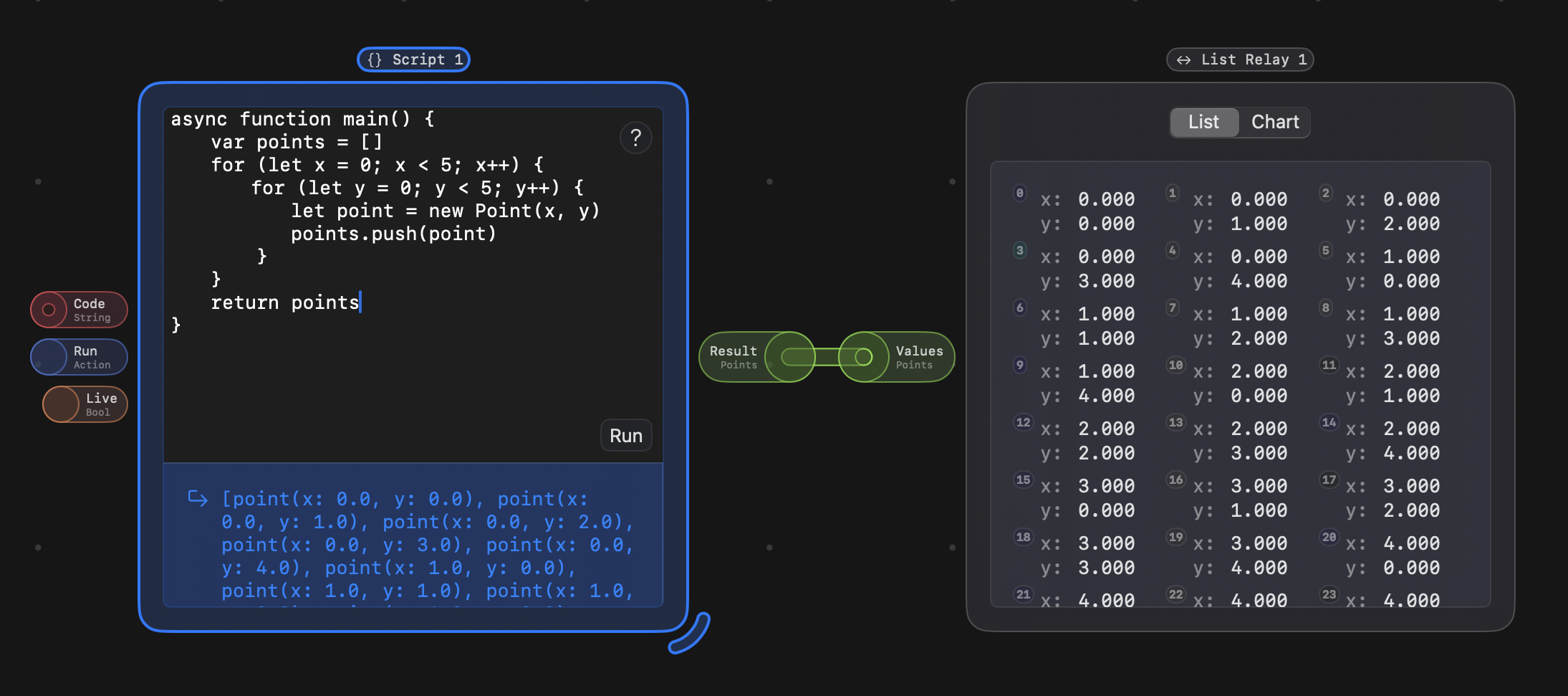
async function main() { var points = [] for (let x = 0; x < 5; x++) { for (let y = 0; y < 5; y++) { let point = new Point(x, y) points.push(point) } } return points }
Nested loops can be used to collect values to return. Here a bunch of Point
types are created in a loop.